Java Sorting and
Searching Program
Algorithm Design · Data Structures · Performance Optimization
Overview
A comprehensive Java-based desktop program that supports reading, editing, sorting, and searching structured data. This project was designed to demonstrate mastery of data structures and algorithmic efficiency.
The goal was to implement a flexible, user-friendly sorting and searching system capable of handling multiple data types (integer, float, string) using various algorithms.
Technology Stack
- Programming Language:
Java 8+ - Core Modules:
Custom ArrayList Collection, Binary Search Tree - Design Tools:
UML & Flowcharts - Project Management:
Timeline planning using Microsoft Project - Benchmarking:
Theoretical and empirical complexity analysis
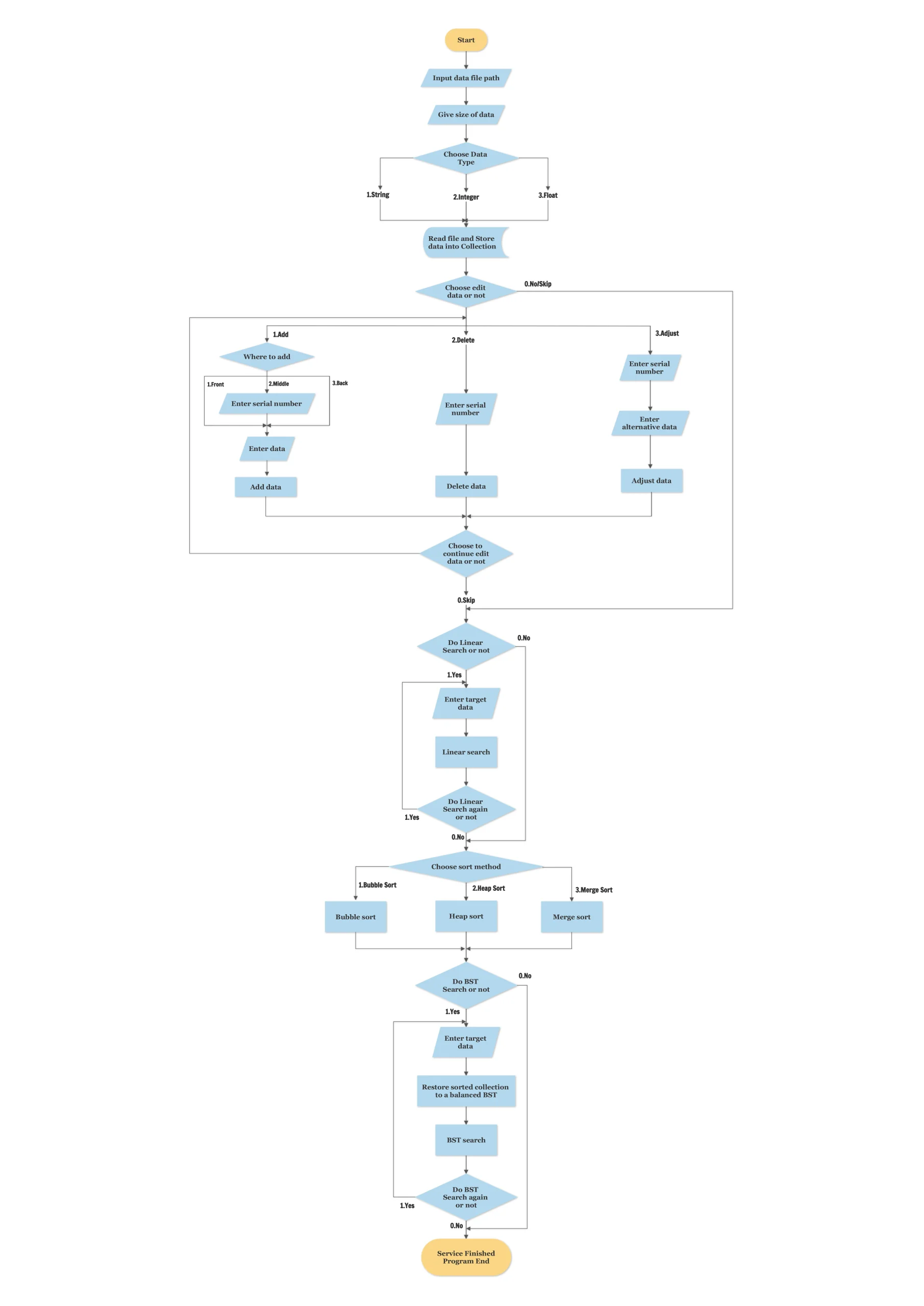
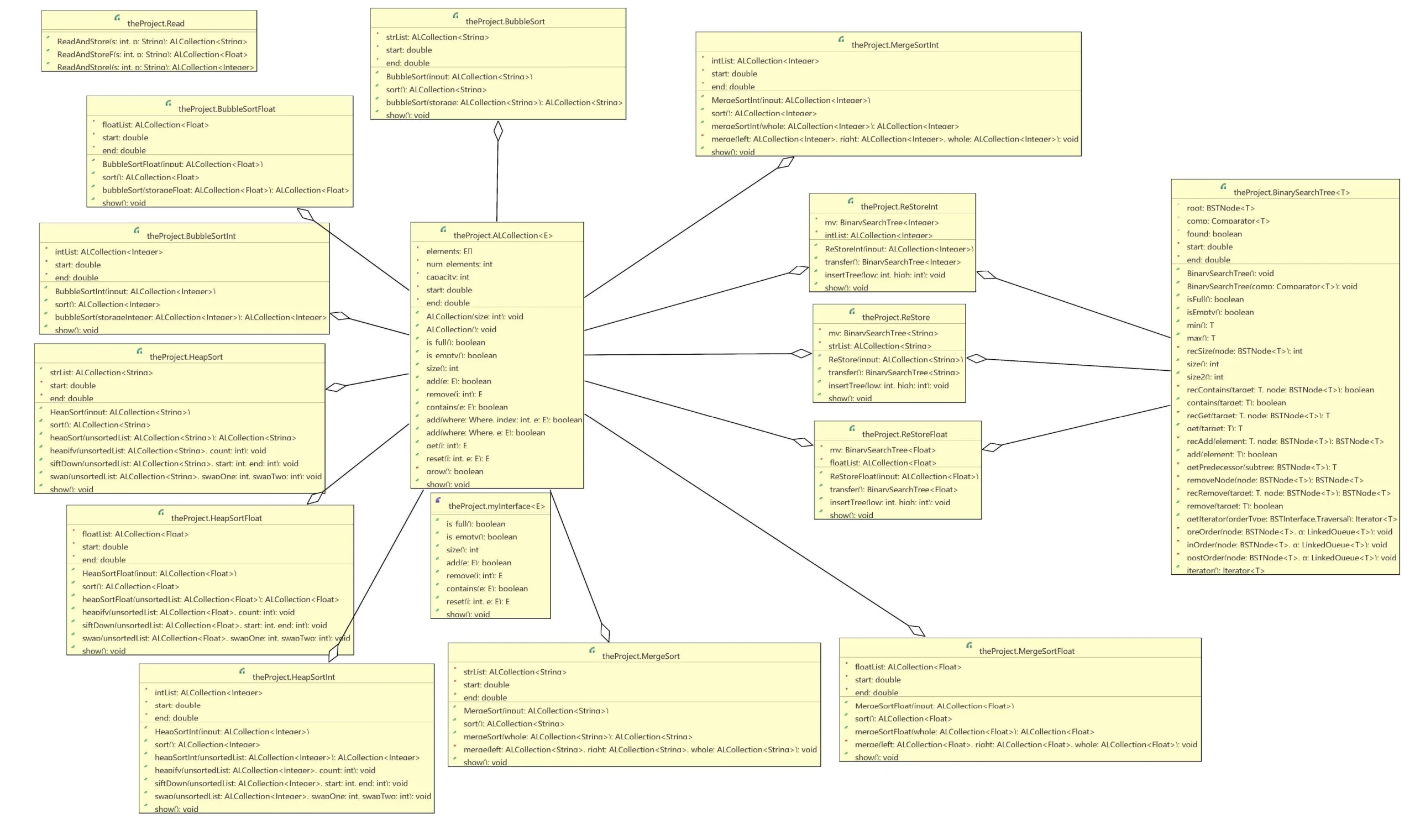
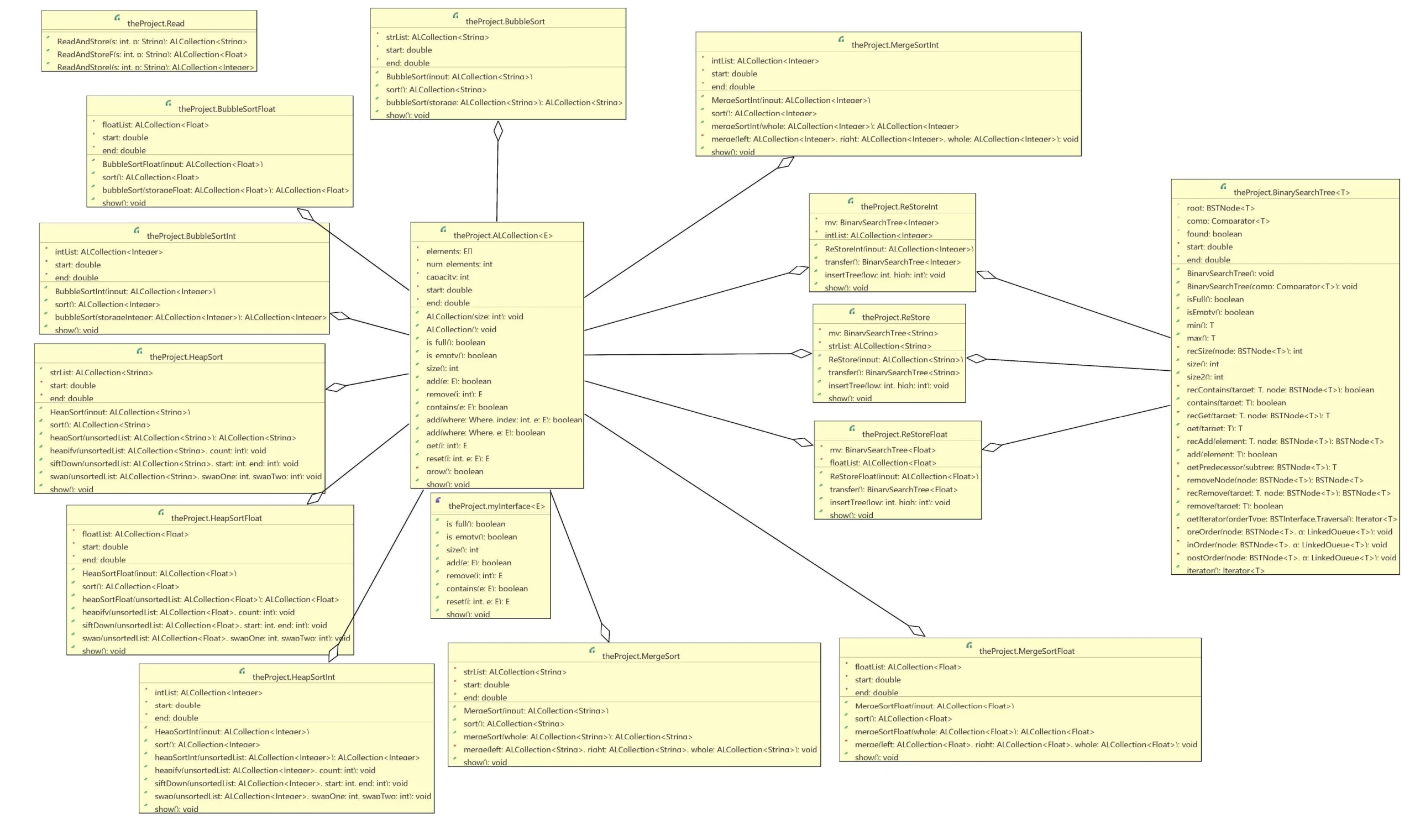
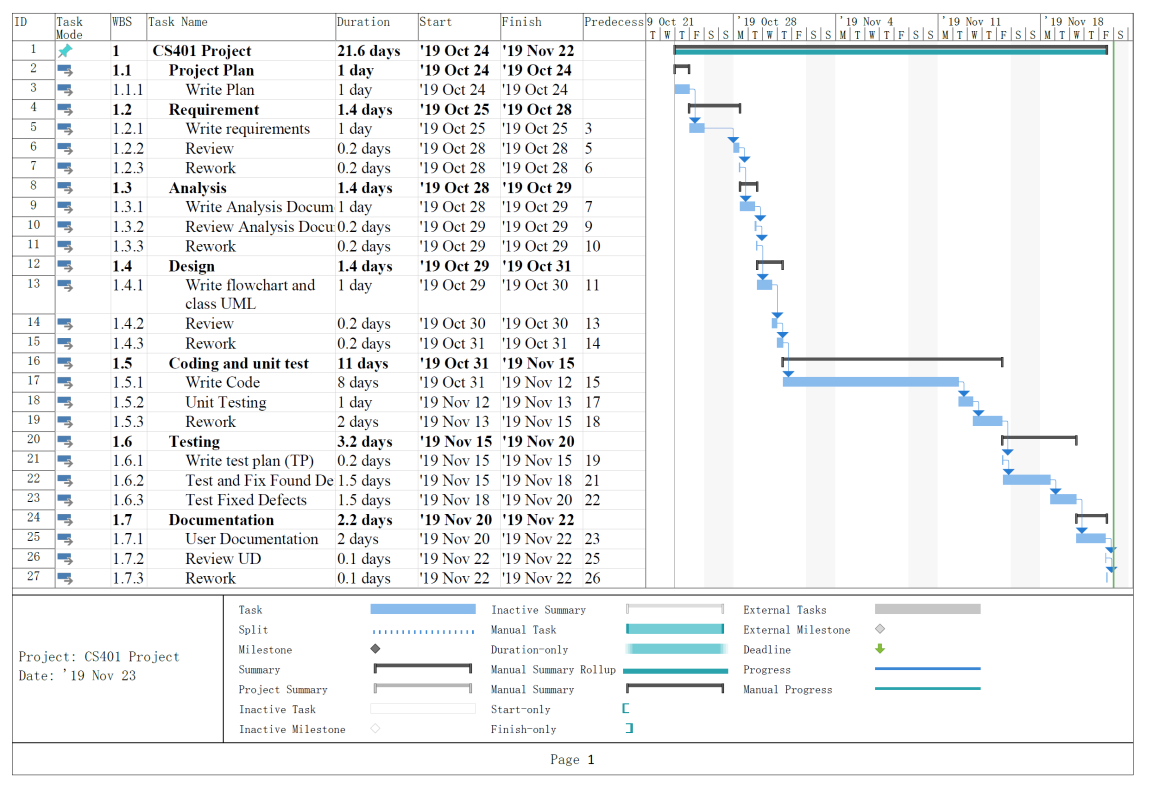
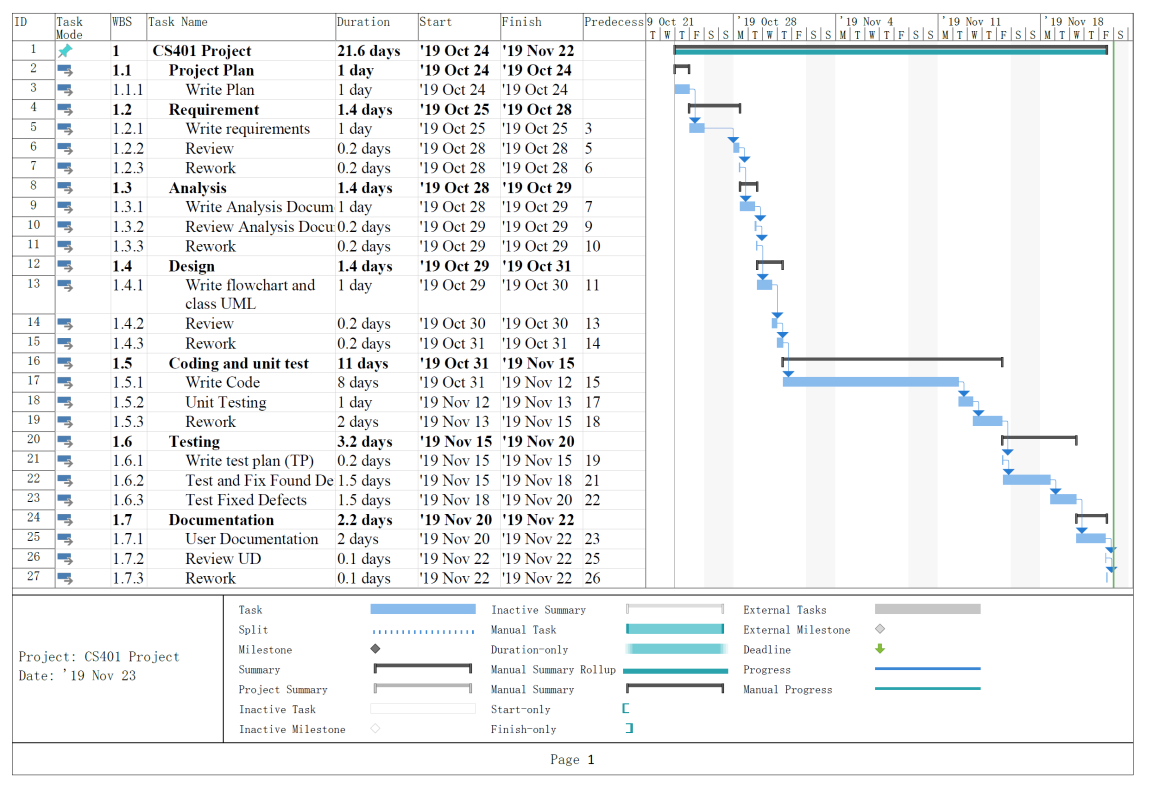
Methodology
and Approach
⚙️ Key Functionalities
- Flexible Data Input
Accepts .txt files with one datum per line. Supports String, Integer, and Float. - Editing Operations
Insert (front, middle, end), delete by index, and modify values. - Sorting Algorithms
Bubble Sort, Heap Sort, Merge Sort. - Searching Mechanisms
Linear Search on unsorted lists, Binary Search via Balanced BST on sorted lists. - Performance Metrics
Nanosecond-level execution time for each sorting and searching operation. - Interactive CLI
Menu-driven user interface allowing repeatable edit-search-sort cycles.
🛠️ Testing Strategy & Automation
- Test Case Generator
Developed a utility to generate random data files across three types and multiple sizes (50–2000 entries), used for testing correctness and performance. - Test Coverage
Validated all operations, including:- Insert/delete edge positions
- Search for missing values
- Sorting empty/already sorted datasets
- BST reconstruction and balance testing
- Performance Benchmarking
Execution time captured via System.nanoTime(); used to produce complexity analysis charts.
Results and Impact
Demonstrated algorithm complexity trade-offs in real-time through runtime comparison. Support for flexible data types and full-featured desktop interaction.
📊 Algorithm Complexity
Algorithm | Best Case | Worst Case |
---|---|---|
Bubble Sort | O(n) | O(n²) |
Heap Sort | O(n log n) | O(n log n) |
Merge Sort | O(n log n) | O(n log n) |
Linear Search | O(1) | O(n) |
BST Search | O(1) | O(log n) |
🧠 Engineering Focus
- Translating foundational data structure algorithms into a usable software product
- Designing extensible, type-agnostic Java modules
- Balancing algorithmic correctness, performance, and user usability
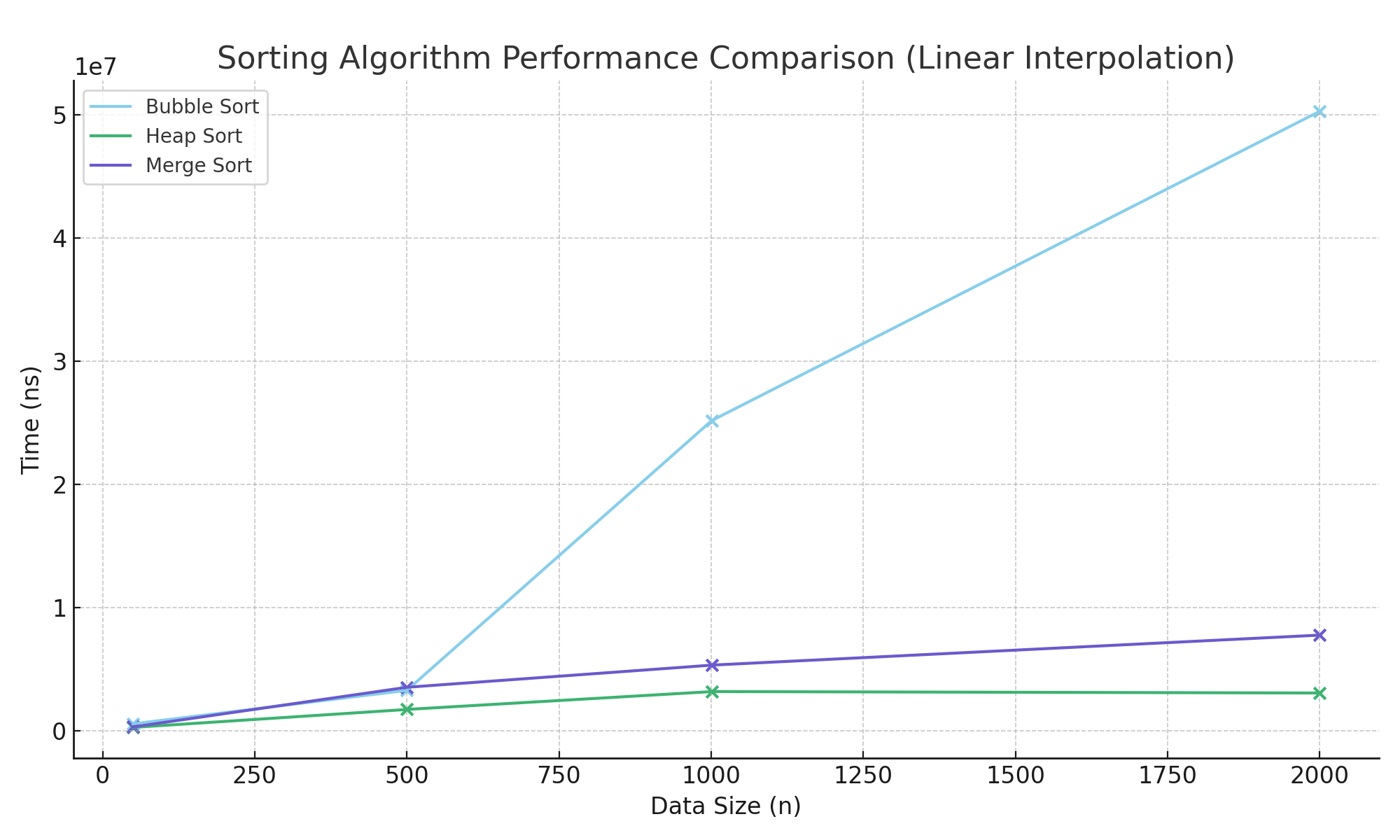
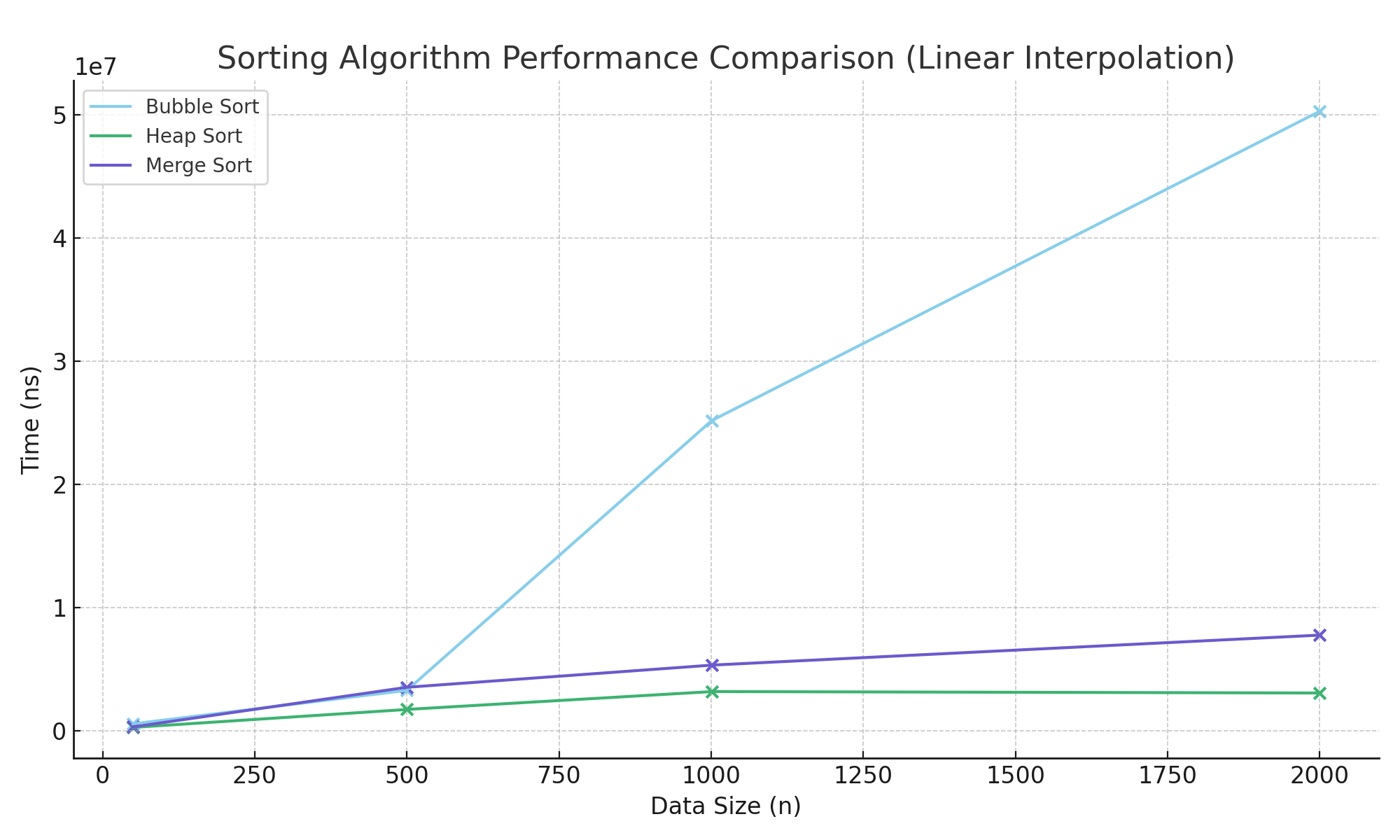
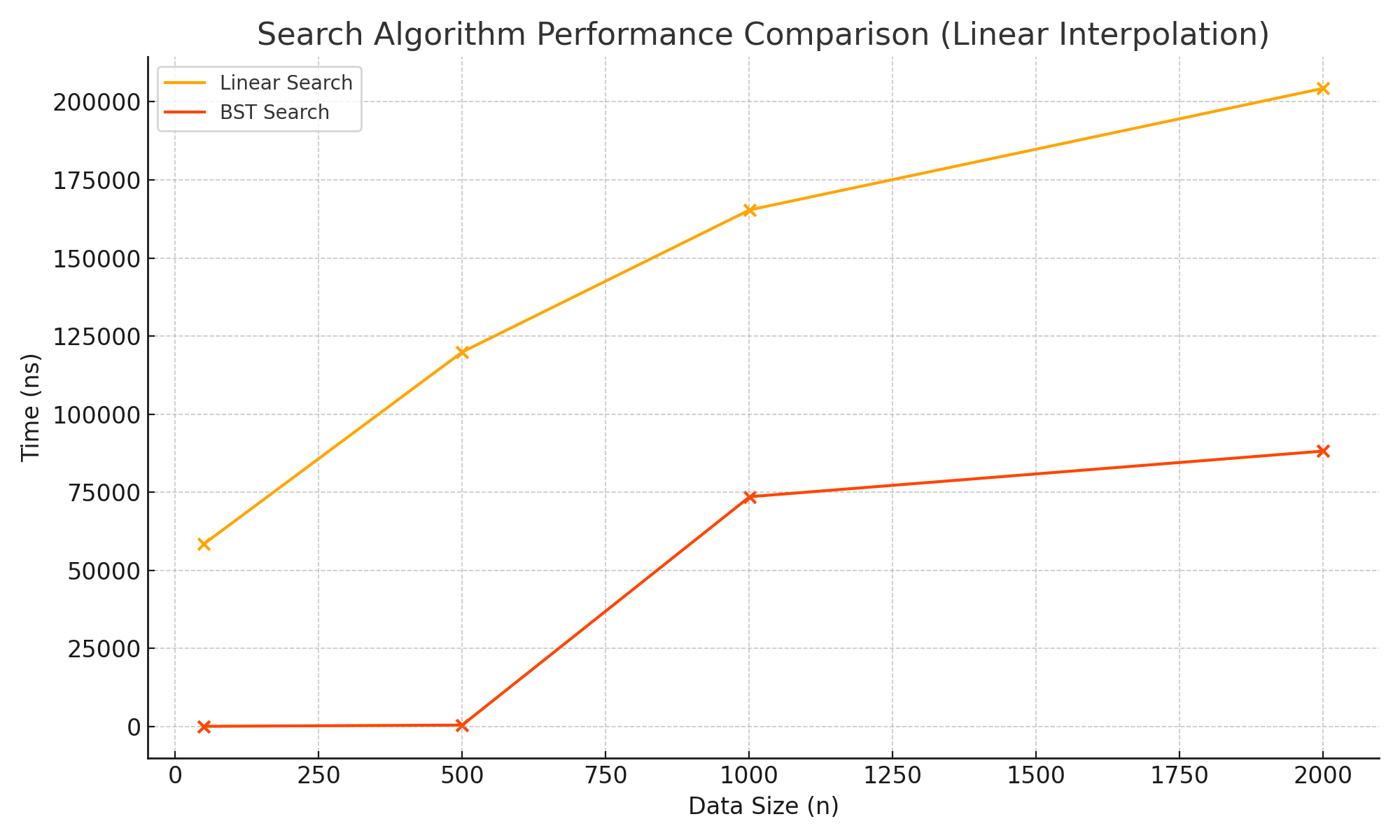
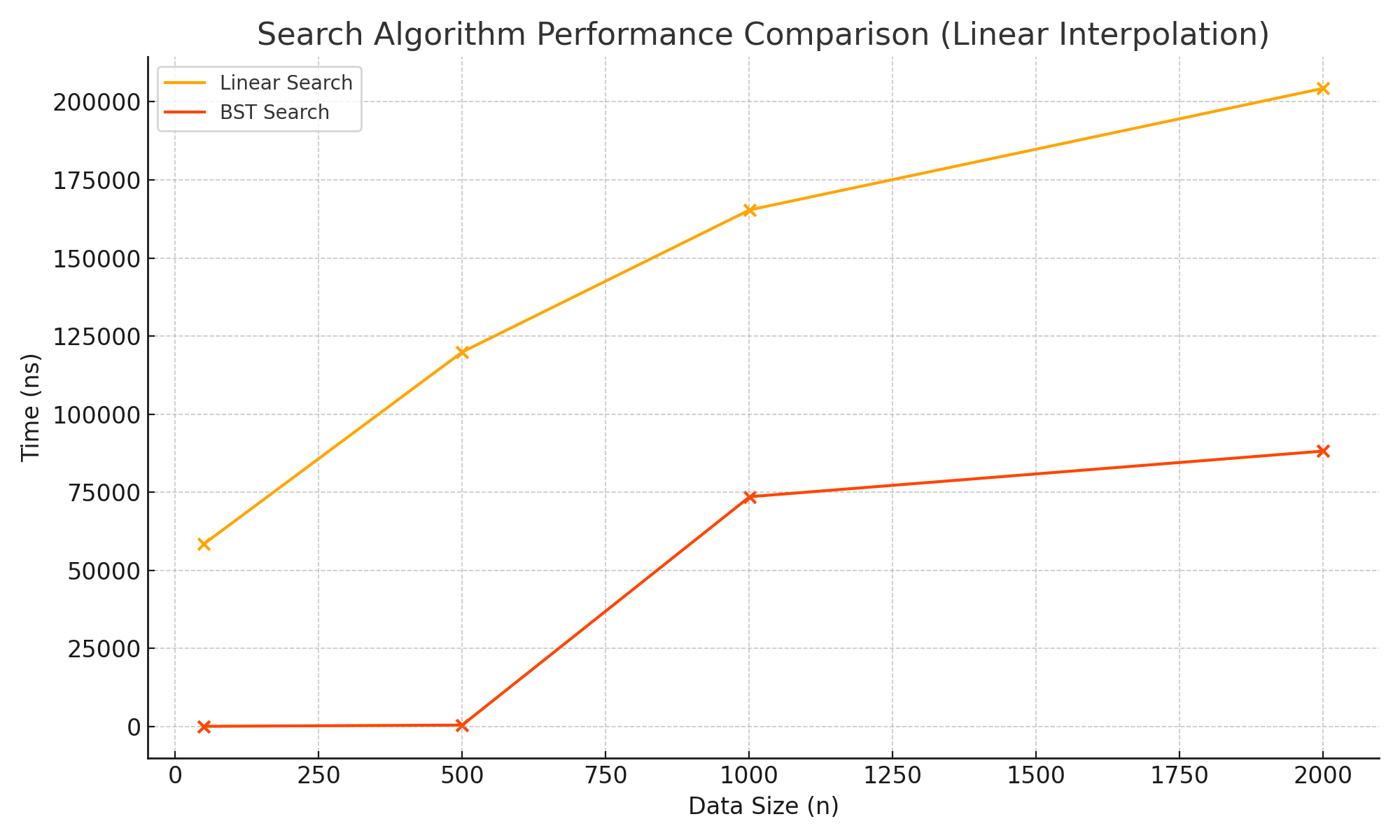
Access Full Details and Files
For full project details, source files, and additional insights, visit the GitHub repository.