Python Programming
Scripting & Automation · Problem Solving · Code Craftsmanship
Overview
This page presents a curated collection of Python programming projects that I developed to systematically strengthen my core software development skills. Each project emphasizes modular program design, top-down design principles, algorithmic thinking, object-oriented development, and simulation techniques, demonstrating a structured and practical approach to solving real-world problems.
Throughout these projects, I explored essential programming domains such as file handling, algorithm optimization (O(n log n)), custom web scraping with HTML parsing, pseudo-random number generation, and Monte Carlo simulations. I focused on writing clear, maintainable, and modular code, applying structured problem-solving strategies to progress from foundational concepts to applied programming practices.
Technology Stack
- Programming Language:
Python 3.x - Core Concepts:
Top-Down Design, Modular Programming, Object-Oriented Design (OOP), File I/O, Web Scraping, Algorithm Optimization, Monte Carlo Simulation - Tools & Libraries:
Standard Python Libraries (HTMLParser, math, random, etc.)
Methodology
and Approach
📚 Featured Projects
Project | Description | Key Skills |
---|---|---|
Stem-and-Leaf Plot Program | Designed and implemented a textual data visualization tool based on a modular top-down design. | Modular Programming, File Handling |
Cups and Dice Game | Built a full object-oriented dice game with multiple dice types, betting mechanics, and balance tracking. | OOP, Class Inheritance, Game Simulation |
Goldbach Deuce | Developed an optimized Two-Sum algorithm with O(n log n) time complexity. | Algorithm Optimization, Binary Search |
Story Plot Generator System | Created a random and interactive story plot generator with MVC-inspired separation. | OOP, MVC Principles |
Surf CDM Web Scraper | Extracted visible text from web pages and performed word frequency analysis using a custom HTML parser. | HTML Parsing, Text Processing |
War and Peace PRNG and Overlapping Ellipses Simulation | Designed a custom PRNG based on literary text and applied it to Monte Carlo simulations. | PRNG Design, Monte Carlo Simulation, 2D Geometry |
🏗️ Key Programming Paradigms and Implementation Strategies
- Top-Down Design: Projects like the Stem-and-Leaf Plot were designed starting with structure charts before code implementation.
- Object-Oriented Programming: Dice Game and Plot Generator were built using class-based inheritance, encapsulation, and polymorphism.
- Algorithm Optimization: Goldbach Deuce project improved brute-force approaches using sorting and binary search.
- Custom Simulation: War and Peace PRNG and Monte Carlo models simulate probabilistic outcomes using real-world text data.
- Text Processing and Web Scraping: HTMLParser was extended to extract and analyze web text for word frequency insights.
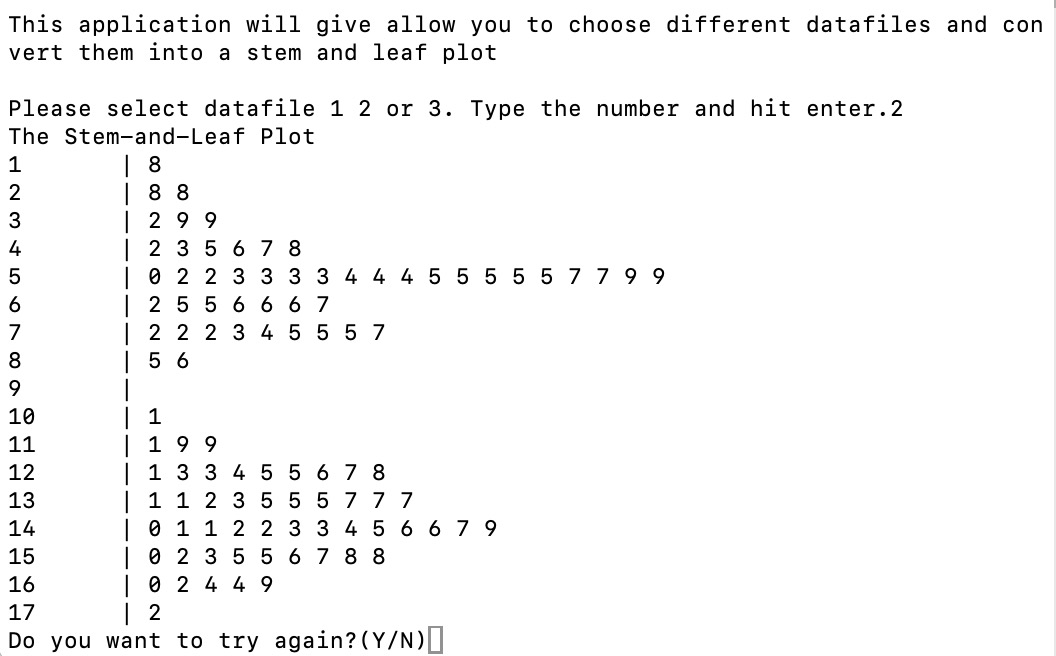
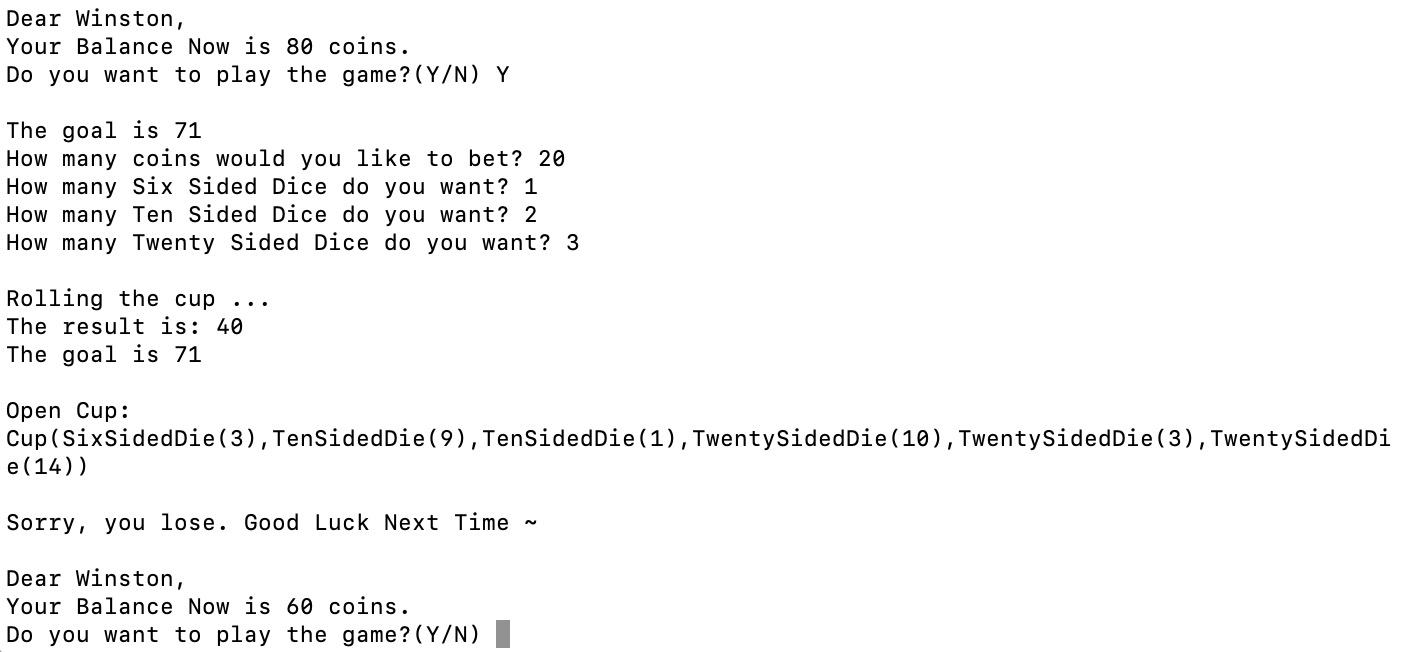
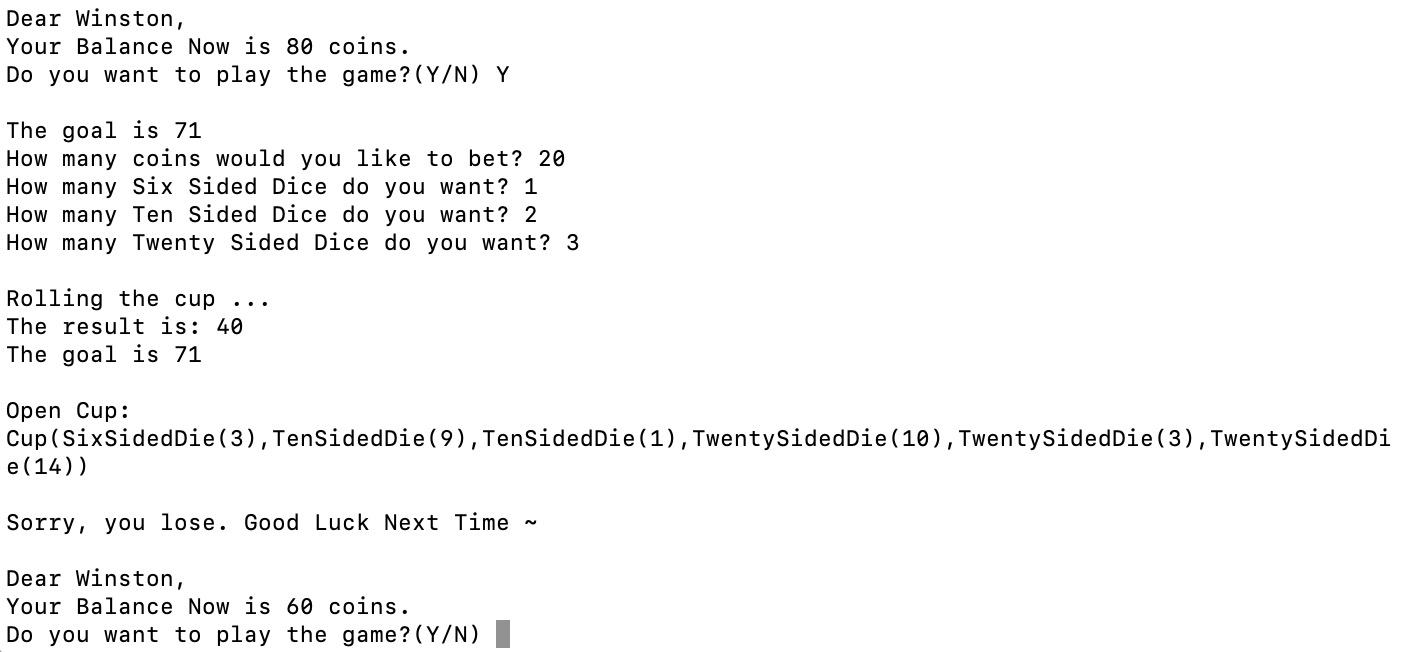
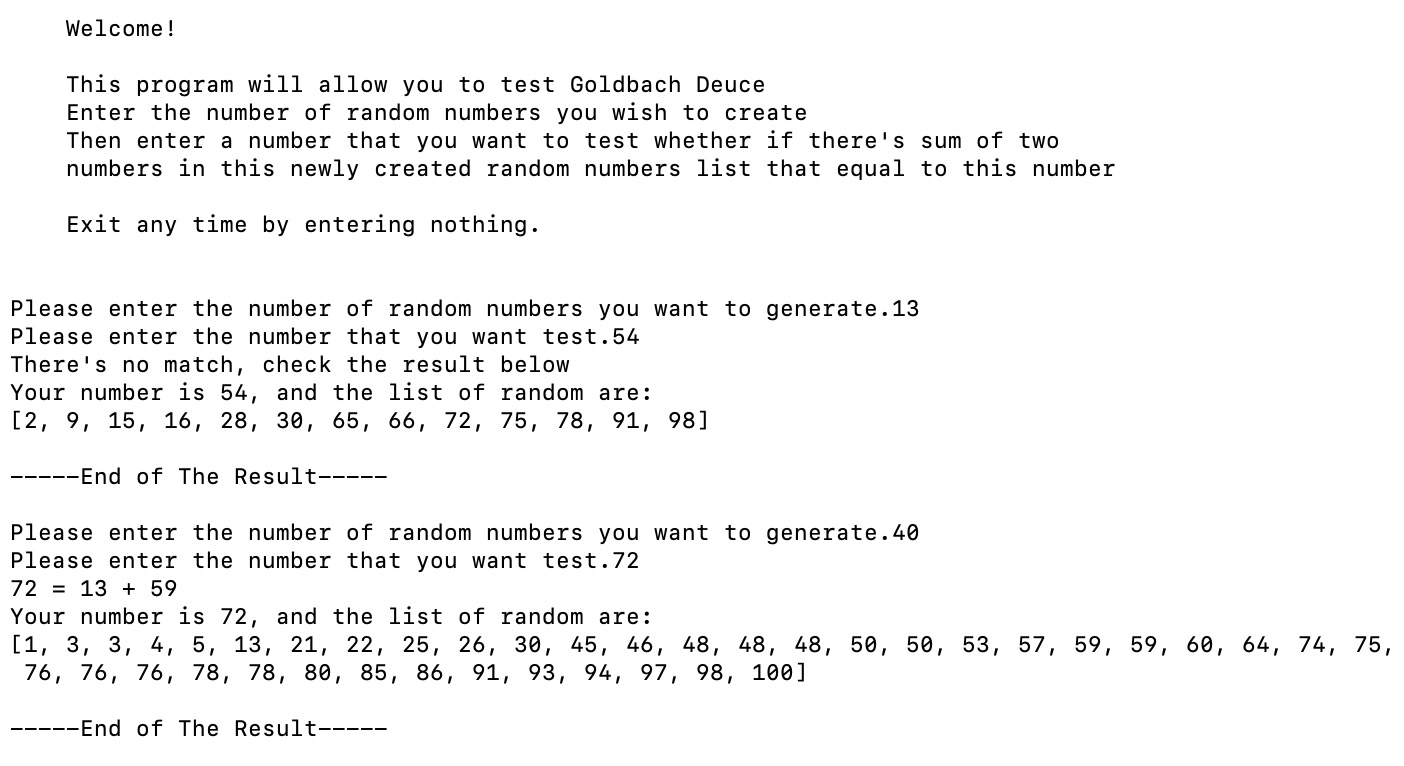
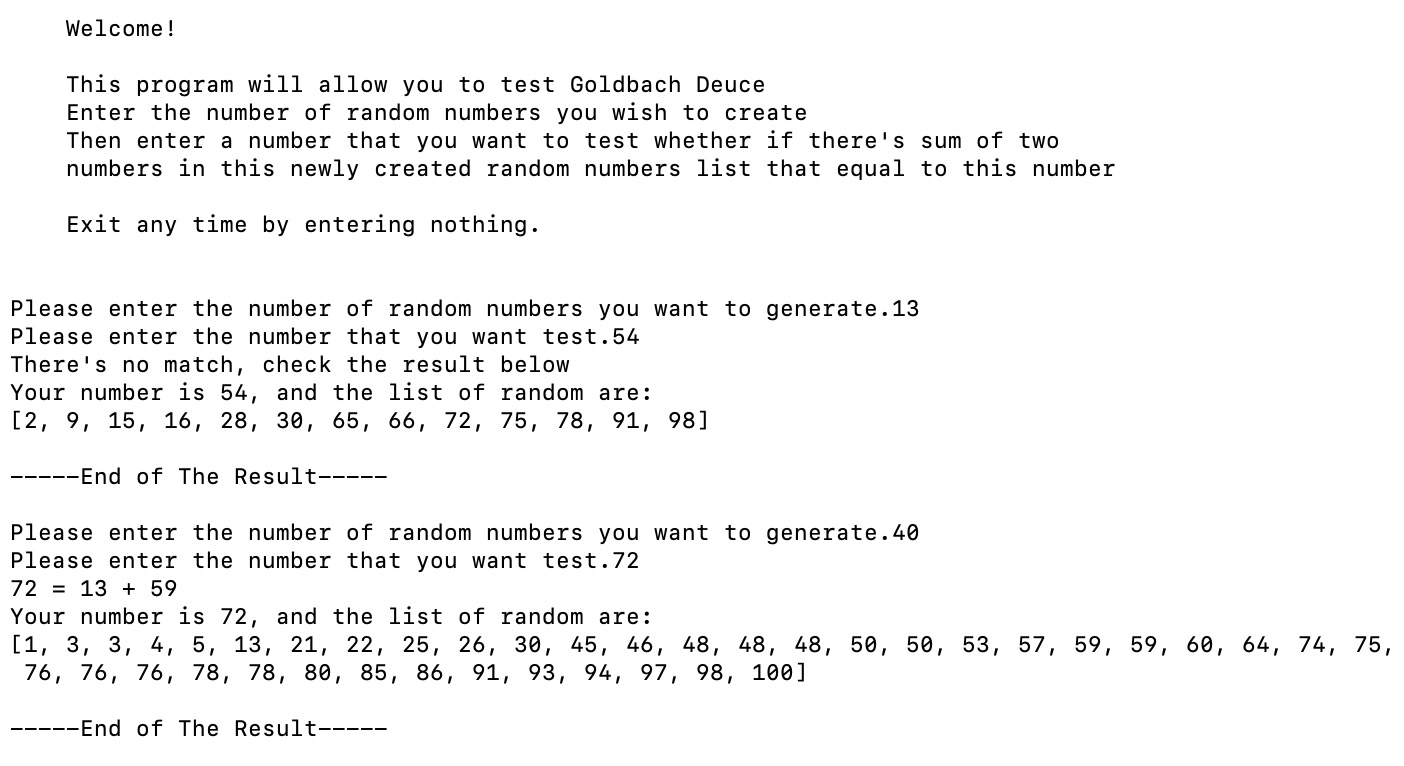
Results and Impact
- Successfully implemented 6+ functional programs combining interactive inputs, custom algorithms, and simulation logic.
- Applied engineering principles (modularization, abstraction, reusability) across all implementations.
- Demonstrated technical growth from structured programming to real-world-inspired simulation and text processing tasks.
- Built a clean, documented, and extensible codebase, uploaded to GitHub with clear project structure and READMEs.
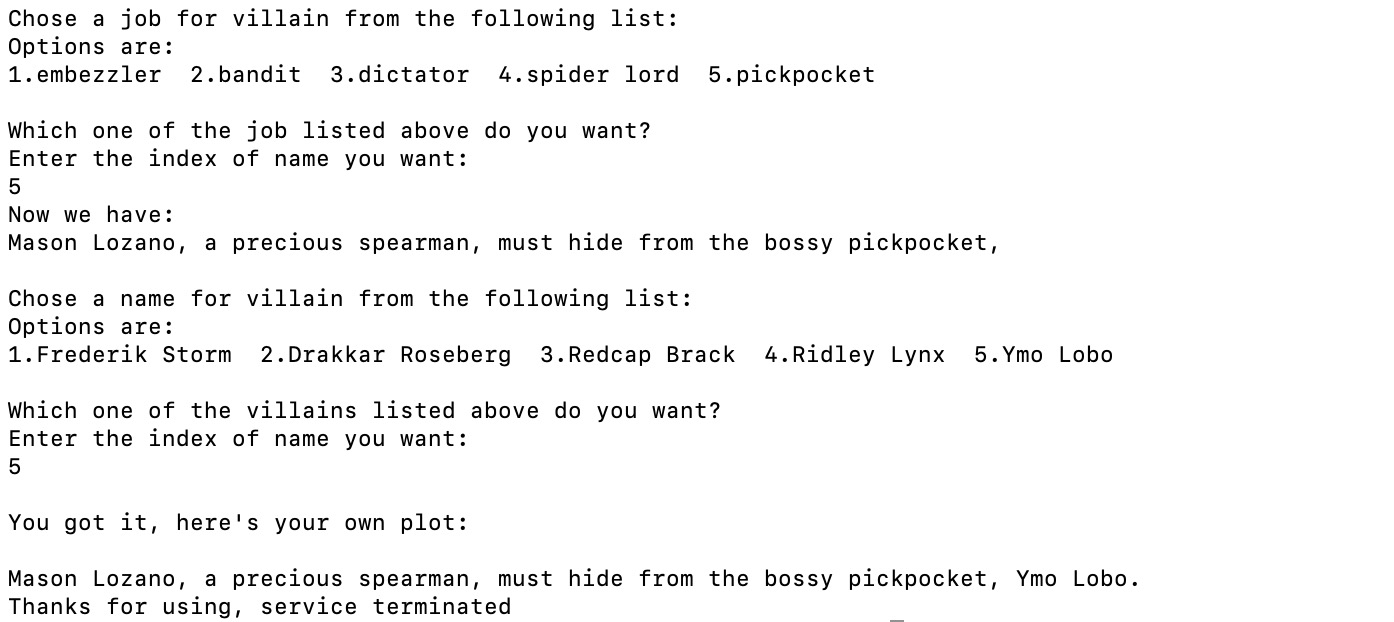
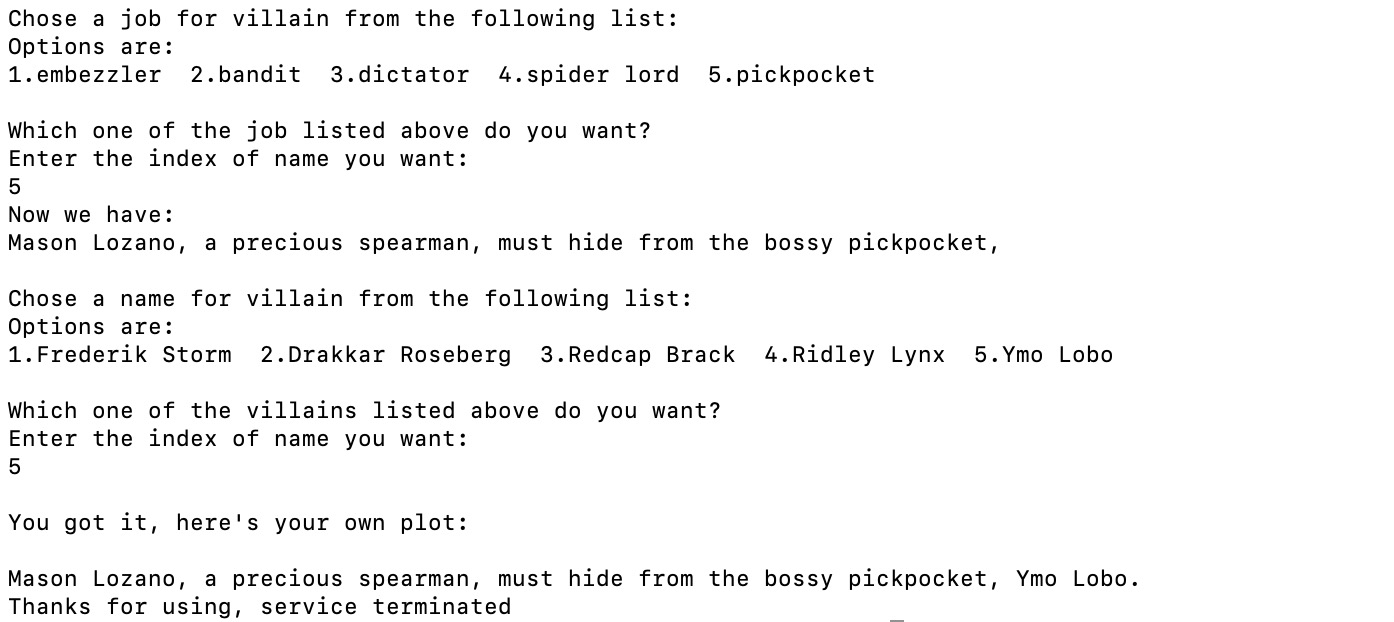
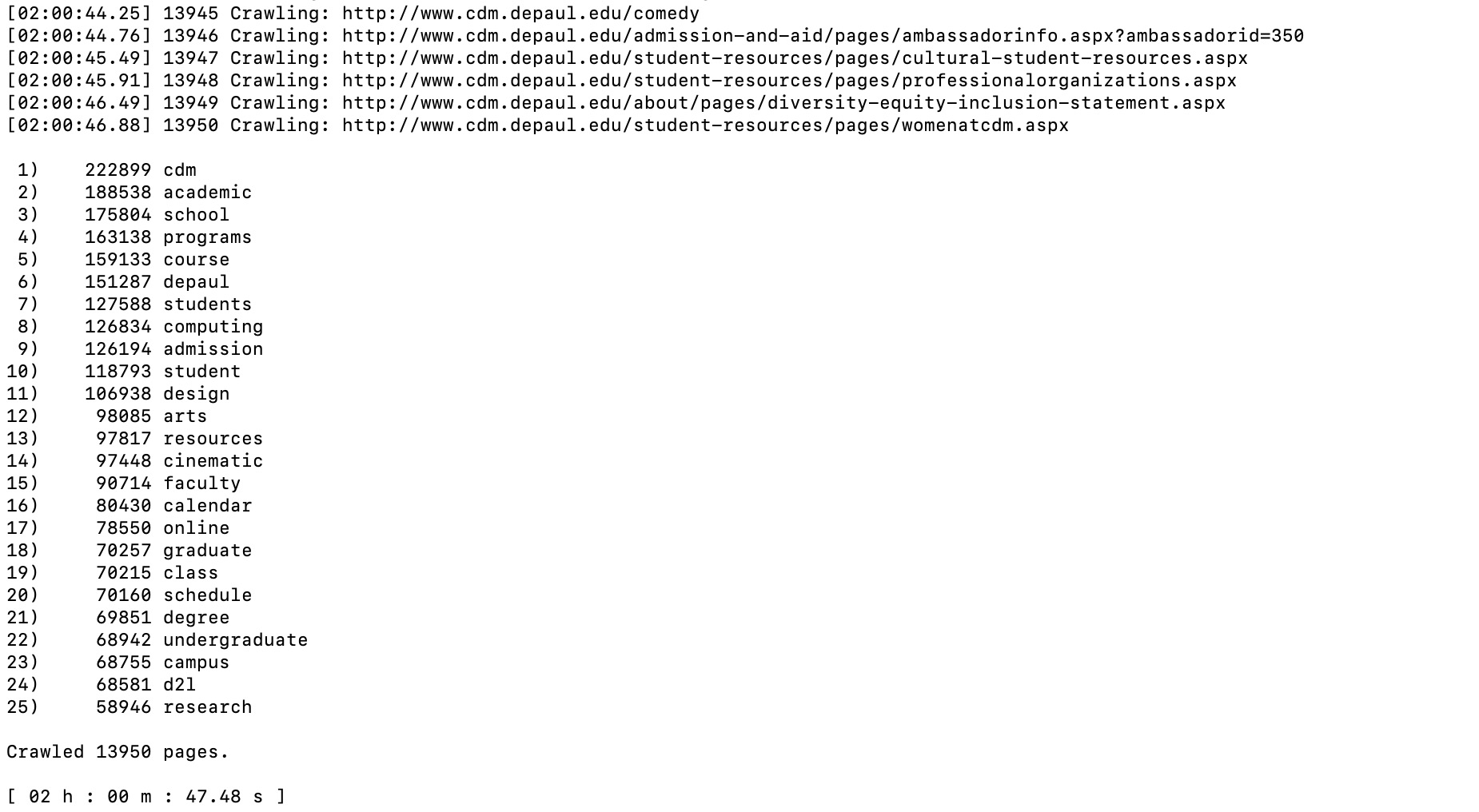
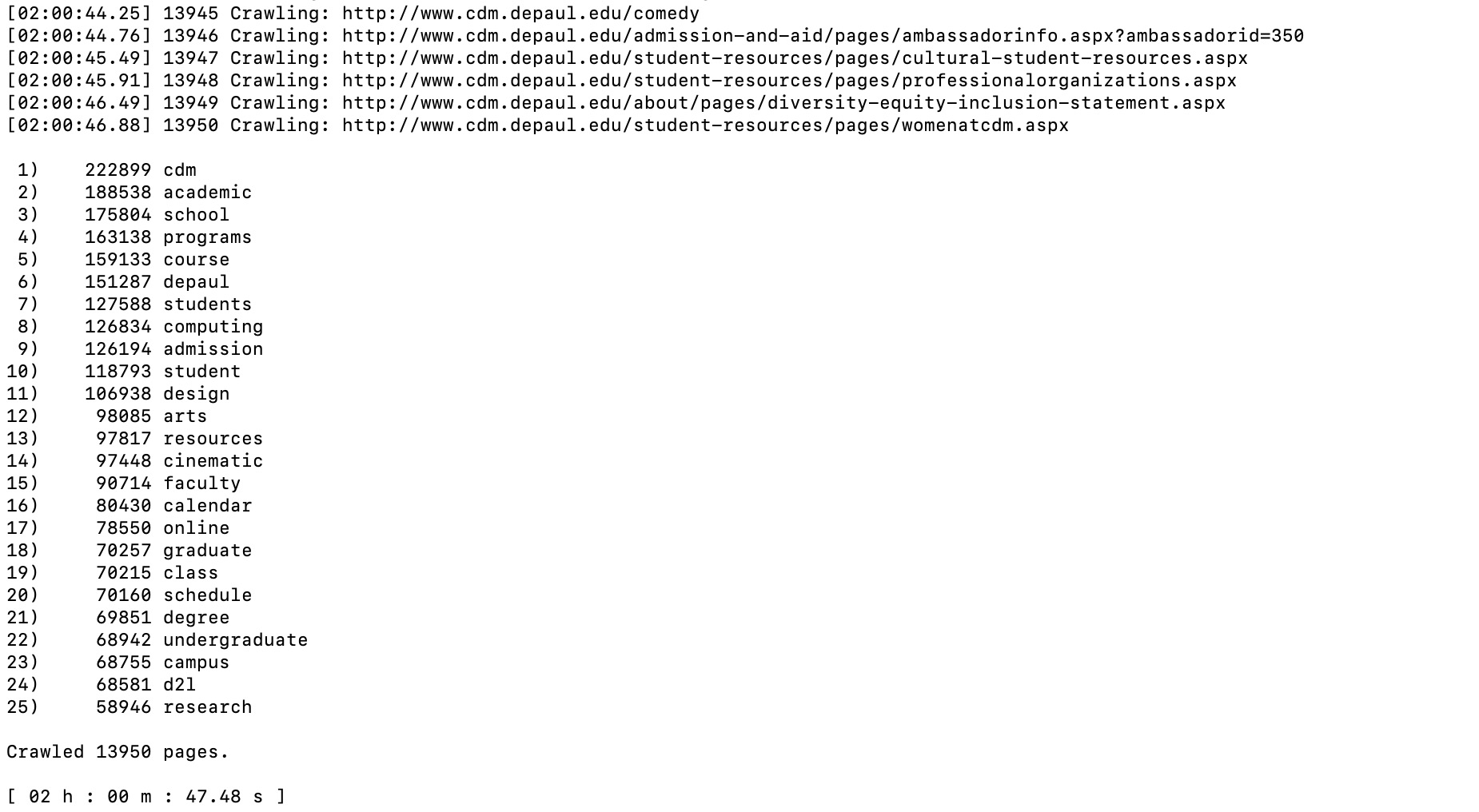
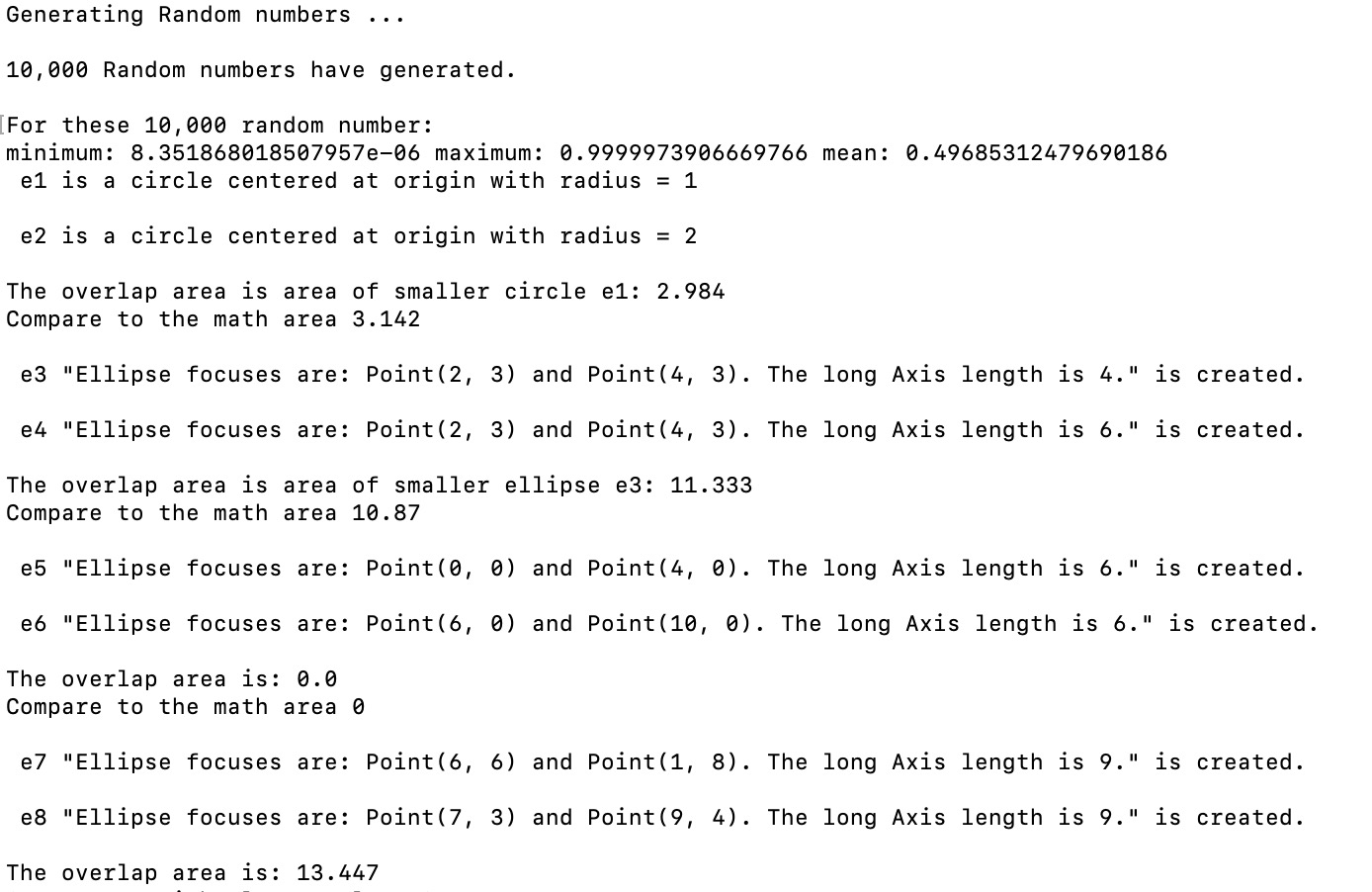
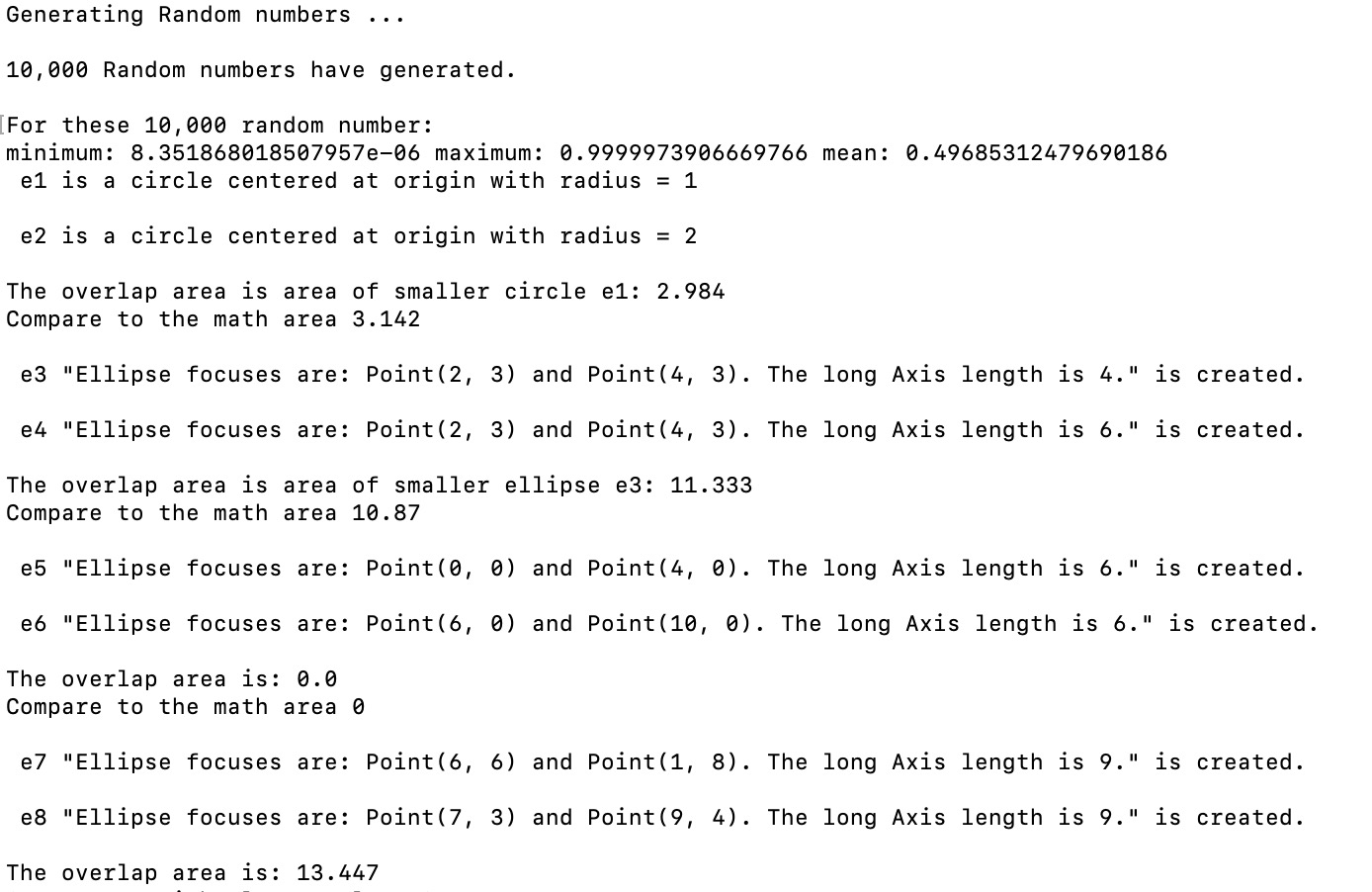
Access Full Details and Files
For full project details, source files, and additional insights, visit the GitHub repository.